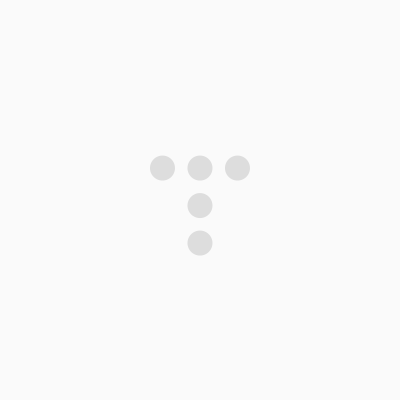
Jackson 라이브러리에서 @JsonProperty(index)를 사용하여 JSON 배열 순서 제어하기JAVA/Java기초2024. 6. 14. 13:25
Table of Contents
Jackson 라이브러리를 사용할 때 JSON 직렬화 및 역직렬화 과정을 제어하는 데 사용된다,.
"@JsonProperty" 의 "index" 속성은 JSON 배열을 처리할 때 각 요소의 순서를 지정하는데 사용된다.
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.ObjectMapper;
class Person {
@JsonProperty(index = 1)
private String name;
@JsonProperty(index = 0)
private int age;
// 기본 생성자 필요
public Person() {}
public Person(int age, String name) {
this.age = age;
this.name = name;
}
// Getter와 Setter 필요
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
public class Main {
public static void main(String[] args) throws Exception {
ObjectMapper objectMapper = new ObjectMapper();
// Person 객체 생성
Person person = new Person(25, "John");
// 객체를 JSON 배열로 직렬화
String jsonArray = objectMapper.writeValueAsString(person);
System.out.println("Serialized JSON Array: " + jsonArray);
// JSON 배열을 객체로 역직렬화
Person deserializedPerson = objectMapper.readValue("[25,\"John\"]", Person.class);
System.out.println("Deserialized Person: Name = " + deserializedPerson.getName() + ", Age = " + deserializedPerson.getAge());
}
}
@JsonProperty(index = 1) ;
"name" 필드가 JSON 배열에서 두 번째 요소임을 지정.
@JsonProperty(index = 0) ;
"age" 필드가 JSON 배열에서 첫 번째 요소임을 지정.
위 코드에서 "Person" 객체는 두 개의 필드를 가지고있고 "name" 과 ''age" 각 필드는 @JsonProperty 어노테이션과 "index" 속성을 사용하여 JSON 배열에서의 위치를 지정한다.
직렬화
- 객체를 JSON 배열로 직렬화할 때 "index" 속성에 따라 순서가 결정된다.
- 결과 "[25, "John"]"
역직렬화
- JSON 배열을 객체로 역직렬화할 때도 "index" 속성에 따라 JSON 배열의 각 요소가 필드에 매핑된다.
예제에서 "[25,\"John\"]" JSON 배열을 역직렬화 하면 'age' 필드는 25로 'name' 필드는 "John" 으로 설정된다.
'JAVA > Java기초' 카테고리의 다른 글
자바에서 SelectList와 SelectOne 이해하기 (0) | 2024.05.28 |
---|---|
자바에서 Map, List 이해하기 (0) | 2024.05.28 |
배열 ( Array) (0) | 2023.09.24 |
프로그래밍 3대 요소 (0) | 2023.07.29 |
JVM과 자바의 구동방식 (0) | 2023.07.24 |
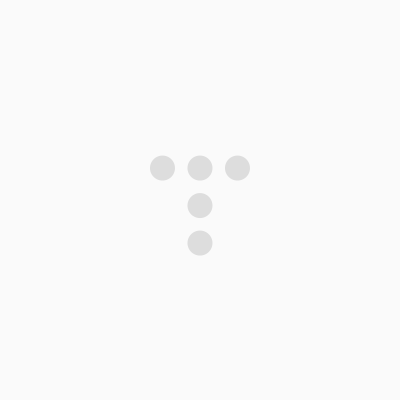
@Soohocoding :: Soohocoding
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!